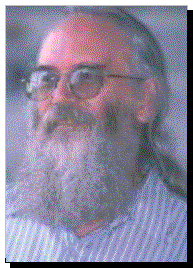
第3回 10月16日 Lecture 3, October 16:
IP Networking Basics
Outline of This Lecture
- Review of last week's homework
- Tools and the Software Development Process
- The TCP/IP Protocol Stack in the Internet
- Internet Protocol addressing (v4/v6)
- Request/response operation
- Datagram v. stream
- Homework
Review of last week's homework
Problem 2 in the homework last week asked you to do the following:
- Above, we defined the function MakeElementList().
Write a program including the
function FreeElementList(), using the library
function free().
Your solution should look something like this:
#include <errno.h>
struct element {
struct element *next;
int elementNumber;
}
struct element *MakeElementList(int n) {
struct element *tmp = malloc(sizeof(struct element));
if (!tmp) {
perror("MakeElementList"); exit(-1);
}
if (!n) {
tmp->next = NULL;
else {
tmp->next = MakeElementList(n-1);
}
tmp->elementNumber = n;
return tmp;
}
void PrintElementList(struct element *in) {
printf("elementNumber: %d\n",in->elementNumber);
if (in->next)
PrintElementList(in->next);
}
void FreeElementList(struct element *in) {
if (in->next)
FreeElementList(in->next);
free(in);
}
main()
{
struct element *mylist;
tmp = MakeElementList(10);
if (!tmp)
exit(-1);
PrintElementList(tmp);
FreeElementList(tmp);
}
Tools and the Software Development Process
We will not have time to go into these topics in detail, but they are
worth mentioning:
- Defining the problem
- Designing your program
- Good design saves implementation and debugging time.
- Design (and code) defensively.
- Creating your program
- Editors
- Source code control
- Building your program
- compiler
- assembler
- linker
- make
- Understanding, fixing and improving your program
- unit tests
- debuggers
- profilers
The TCP/IP Protocol Stack in the Internet
"Network programming" is a broad title. It could refer to any
networking protocol: DECNET, SNA, OSI, ATM. In this class, we will
focus only on Internet Protocol (IP) networking, and the TCP/IP suite
of related protocols built around it.
From Wikipedia:
Internet Protocol addressing (v4/v6)
The Internet is known as a packet switched network, rather
than circuit switched. Data on the Internet is transferred
in IP packets. Each packet is identified by its source
and its destination. Normally, the source and destination
identifiers each contain:
- Address (IPv4 or IPv6)
- Upper-Layer Protocol identifier (e.g., TCP or UDP)
- Port number (if ULP is TCP or UDP)
The lowest layer we will concern ourselves with (for the moment, at
least) is the network layer, which in our case is
the Internet protocol. From Wikipedia, the IPv4 packet
header:
And the IPv6 packet header:
After the IP header (whether v4 or v6), comes
the transport-layer header. The only part of the
transport-layer header we are interested in at the moment is
the port number.
Request/Response (Client/Server) Operation
Most Internet-based applications behave in a request/response
manner. Typically, though not always, requests are small, and
responses are large. Most of the time, the requestor is
a client, such as regular PC, and the responder is
a server, such as a web server.
Datagram versus stream
When the response to a request is expected to be long, an application
will usually want to open a stream. For simple, short
requests, a datagram may be good enough. We must learn how to
do both types of network operations.
宿題
Homework
This week's homework (submit via email):
- Last week, you were supposed to create three source files and link
them together for your program. This week, we are going to
add unit test. We need two more source code files:
- mylibtest.c
- networkingtest.c
Each of those should include a main() function that
calls each function in the corresponding source file to test
that it works. Now you must compile three separate programs:
hotpotato, mylibtest, and networkingtest. Show that each one runs.
- The homework "answer" at the top of this page does not actually
work. Please copy that to your computer, and debug it. (You may
also want to use this as an opportunity to try a source code control
system, such as RCS, and my preference is that you send me
the solution to this problem as a diff or rcsdiff from
the existing program.)
Next Lecture
第4回 10月23日
Lecture 4, October 23: Basic socket programming 1
Additional Information
その他